This homework aims to establish a stronger understanding of how we can manipulate user inputs and a general understanding of request methods (POST in this case).
Step 1
Create a folder inside public with the name homework01. Inside homework01 folder create index.php with following code and copy.
<h2>Pancake Calculator</h2>
<form method="post">
<p>
How many pancake you want? <input type="number" name="amount" />
</p>
<input type="submit" value="Calculate" />
</form>
<?php
if (!empty($_POST)) {
echo '<h3>You need</h3>';
echo '1000g Flour <br/>';
echo '20 Eggs <br/>';
}
?>
<p>
For 10 pancakes we need:
<ul>
<li>100g flour</li>
<li>2 eggs</li>
<li>300ml milk</li>
<li>1 tbsp oil</li>
</ul>
</p>
Everything should look like picture below.
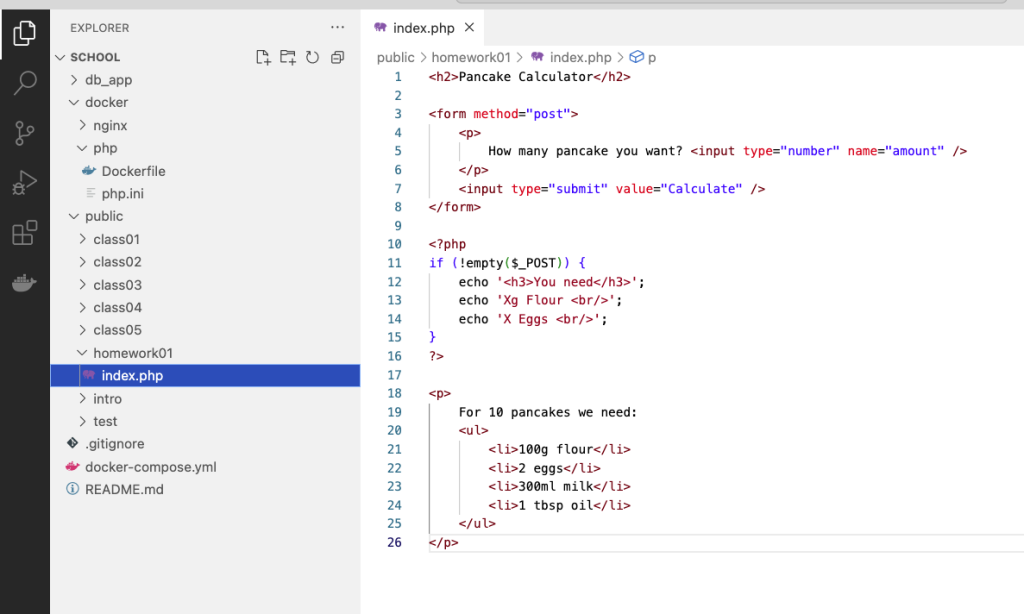
Step 2
Open homework01.localhost in your browser, and you should see a simple HTML page with one text input and a pancake recipe below.
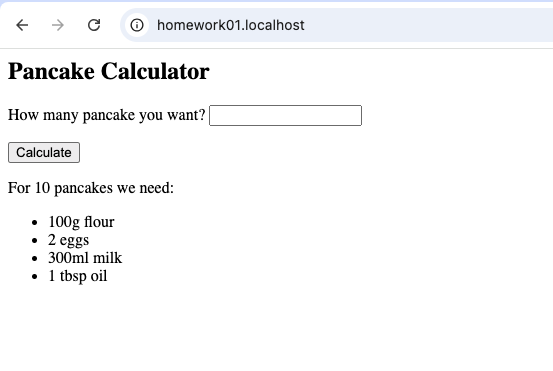
Step 3
If you type any number of pancakes in the field and click “Calculate”, you will get a response on how much Flour and how many Eggs you need for it.
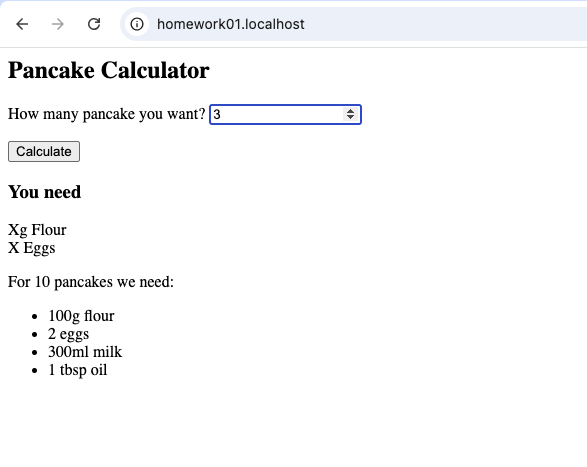
You got that response because we have code that checks if the POST params are empty or not.
if (!empty($_POST)) {
Task
Unfortunately response is always X and never the real value. Your task is to show the actual amount of Flour and Eggs you need for the given number of pancakes.
You can check the recipe values for reference. For example, if the user wants 100 pancakes, we will need 1000g of Flour and 20 Eggs (like in picture bellow). Feel free to show the results in whatever units of measurement you like.
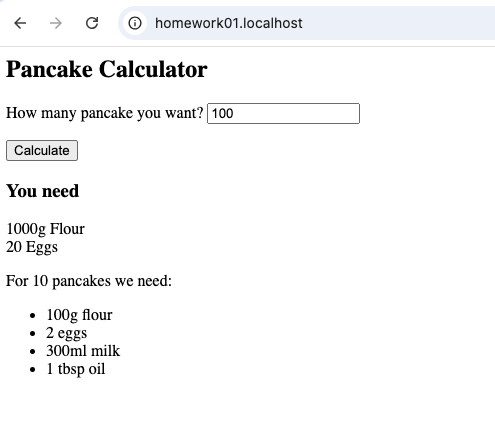
If you feel extra creative, display how much Milk and oil is needed as well 🙂